Picture this: You’re trying to access your favorite website to check something important, but the internet connection is spotty or drops altogether. Now, normally you’d get frustrated with an “offline” message staring you in the face. But imagine if the website worked perfectly even without a connection. You could still scroll through content, click buttons, or even submit forms. That’s the magic of Progressive Web Apps (PWAs) or PWA Progressive Web App —they behave like native apps, but they live on the web.
If you’re wondering, What is a PWA progressive Web App? A PWA is essentially a website that feels like an app. By leveraging modern web technologies, PWAs can work offline, load quickly, and even be installed on your phone or desktop. Unlike regular websites, they offer a better user experience by giving the impression of being native apps, but without the fuss of downloading them from an app store.
In this guide, we’ll dive deep into What a PWA Progressive Web App is, why it’s important, and—most importantly—how you can build your own PWA in just five steps. By the end of this article, you’ll have a clear understanding of how to implement advanced caching strategies and service workers to create a fully functional, offline-capable PWA. So, buckle up!
What is a PWA Progressive Web App (PWA)?
Before we jump into the building process, let’s take a step back and really understand what a PWA is. At its core, a PWA is a web application that uses modern web capabilities to deliver an app-like experience to users. Think of it as the best of both worlds: the convenience of web apps and the reliability of native apps.
Here’s why PWAs are a big deal:
- Offline Mode: With the help of caching strategies and service workers, PWAs can function offline. Whether you’re stuck in an elevator with no signal or your Wi-Fi is acting up, PWAs make sure the user experience is uninterrupted.
- Installable: Users can install a PWA on their devices directly from their browsers without the hassle of going through app stores.
- Cross-Platform: PWAs work across devices (phones, tablets, desktops) and on different operating systems like Android and iOS.
- Improved Performance: PWAs load content faster, which is critical for retaining users in today’s fast-paced digital world.
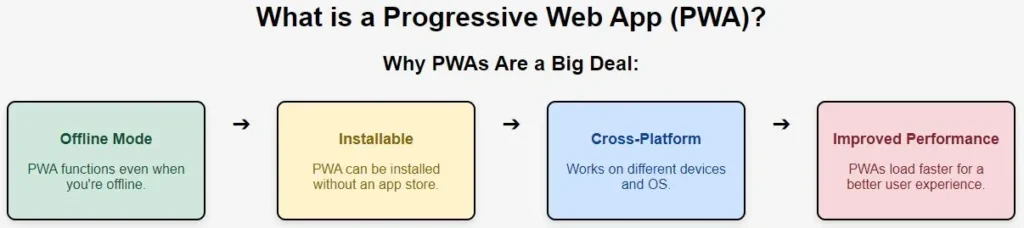
PWA vs. Native Apps:
You might be wondering, “Why not just build a native app instead?” That’s a fair question. Let’s compare PWAs with native apps in a quick table:
Feature | PWA | Native App |
---|---|---|
Installation | Installable via the browser | Requires App Store or Play Store |
Cross-platform | Works on all devices via browser | Separate builds for Android & iOS |
Offline functionality | Yes, with caching | Yes |
App Store approval | Not required | Required |
Development cost | Lower, single codebase | Higher, separate codebases |
Access to device features | Limited (e.g., Bluetooth access) | Full access |
In short, PWAs offer a more accessible, less expensive alternative to native apps, but they may lack access to some advanced device functionalities. Still, for most use cases, PWAs are an excellent solution.
Why PWA App Are Important
We’ve touched on this briefly, but let’s dig deeper into why PWAs are essential for modern businesses and developers.
1. Offline Access:
Ever used a native app that lets you browse content offline? PWAs achieve the same functionality. By using service workers and advanced caching techniques, a PWA can store critical assets like HTML, CSS, JavaScript, and images locally on a user’s device. This means that even when the user loses internet connectivity, the app continues to function with cached data. It’s like having your website live in the user’s pocket.
2. Fast Loading Times:
Nothing drives users away faster than a slow website. According to Google, 53% of users leave a site that takes longer than 3 seconds to load. With a PWA, you can significantly reduce loading times through efficient caching, preloading, and other techniques.
3. Enhanced Engagement:
PWAs can send push notifications to users, similar to native apps. This allows businesses to re-engage users and drive conversions. Additionally, because PWAs can be installed on a user’s device, they’re always just one click away, which improves user retention.
4. Cost-Effective:
Developing a PWA is often cheaper than building native apps for both iOS and Android. With a PWA, you write code once and deploy it everywhere, without worrying about the extra costs associated with maintaining separate codebases for different platforms.
Building a Progressive Web App in 5 Simple Steps
Now, let’s get to the meat of the article: building a PWA in five simple steps. The best part? You don’t need to be a seasoned developer to follow along. By the end of this section, you’ll have a fully functional, installable PWA that works offline.
Step 1: Create the Basic Web App
Start by creating a basic web app using HTML, CSS, and JavaScript. This will act as the foundation for your PWA. Don’t overcomplicate things—your app can be as simple or as complex as you like.
Example folder structure:
index.html
main.css
app.js
Basic index.html
template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My PWA</title>
<link rel="stylesheet" href="main.css">
</head>
<body>
<h1>Welcome to My PWA</h1>
<p>This is a basic web app that we’ll convert into a PWA.</p>
<script src="app.js"></script>
</body>
</html>
At this stage, you’re just laying the groundwork for the more exciting stuff. This web app will evolve into a PWA once we add a few more features.
Step 2: Add a Web App Manifest
The Web App Manifest is a JSON file that provides metadata about your web app. This includes things like the app’s name, icons, background color, and start URL. The manifest is crucial for transforming your web app into a PWA.
Here’s what a typical manifest.json
looks like:
{
"name": "My Awesome PWA",
"short_name": "PWA",
"start_url": "/index.html",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"icons": [
{
"src": "/images/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "/images/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Once you’ve created your manifest.json
file, link to it from the index.html
file like this:
<link rel="manifest" href="manifest.json">
Now, when users visit your site, their browsers will recognize it as a PWA and provide an option to install it.
Step 3: Register a Service Worker
The service worker is the backbone of a PWA. It’s a script that your browser runs in the background to manage caching and handle network requests. Think of it as a little worker bee making sure your app continues to function smoothly even when users are offline.
Here’s how you register a service worker in app.js
:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
})
.catch(error => {
console.error('Service Worker registration failed:', error);
});
}
This script checks if the browser supports service workers and, if so, registers one. Service workers are essential for offline functionality and can dramatically improve load times by caching resources.
Step 4: Implement Caching for Offline Use
Now let’s get to the fun part: caching assets so that your app can work offline. In your service-worker.js
file, you’ll need to implement a basic caching strategy. Here’s an example:
const CACHE_NAME = 'my-pwa-cache-v1';
const urlsToCache = [
'/',
'/index.html',
'/main.css',
'/app.js'
];
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => {
console.log('Opened cache');
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => {
if (response) {
return response; // Return the cached version
}
return fetch(event.request); // Fetch from network if not in cache
})
);
});
In this example, we’re caching some basic files (like index.html
, main.css
, and app.js
) during the service worker’s installation phase. Then, we intercept network requests and serve cached content if it’s available. If not, we fetch fresh content from the network.
Step 5: Test and Install the PWA
The final step is to test your PWA. Open your app in a browser (Chrome and Firefox are great options) and inspect it using DevTools.
To simulate offline mode, follow these steps in Chrome:
- Right-click anywhere on the page and click Inspect.
- Go to the Application tab.
- Under Service Workers, check the box that says Offline.
- Reload the page and see how your app works offline.
If everything looks good, congratulations—you’ve built a fully functional PWA! Now, when users visit your site, they’ll be able to install it directly onto their home screen.
Advanced Caching Strategies for PWAs
Caching can get a little more complex depending on the nature of your app. Let’s discuss a few advanced caching strategies that go beyond the basics and provide a more seamless experience for users.
1. Cache First, Then Network:
In this strategy, the service worker first tries to serve the cached response. If the resource is not in the cache, it fetches it from the network.
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => {
return response || fetch(event.request);
})
);
});
This approach works well when you have a lot of static resources (like images or CSS files) that don’t change frequently.
Network First, Then Cache:
This strategy prioritizes the network response. If the network is unavailable (such as when the user is offline), it falls back on the cached version.
self.addEventListener('fetch', event => {
event.respondWith(
fetch(event.request).catch(() => caches.match(event.request))
);
});
This is useful when you want to ensure the user gets the most up-to-date data, but still want to provide an offline experience.
Cache and Network Race:
This is a more complex approach where both the cache and the network are accessed simultaneously, and whichever responds first wins.
self.addEventListener('fetch', event => {
event.respondWith(
Promise.race([
caches.match(event.request),
fetch(event.request)
])
);
});
This method can reduce loading times in some situations by serving cached resources quickly while still attempting to fetch fresh content.
Case Study: Twitter Lite’s Success with PWA
One of the most popular examples of a successful PWA is Twitter Lite. Twitter introduced a PWA to solve the problem of high data usage and slow loading times in areas with poor internet connectivity. Here’s what they achieved with their PWA:
- 65% increase in pages per session.
- 75% more Tweets sent.
- 20% decrease in bounce rate.
- The size of the Twitter Lite app was only 600KB, a fraction of their native app sizes.
These results show the power of PWAs in improving user engagement and reducing resource usage.
Pros and Cons of PWAs
Like any technology, PWAs come with their own set of advantages and limitations. Let’s take a balanced look at both.
Pros of PWAs:
Works Offline: PWAs can store data in the browser’s cache, allowing them to work even without an internet connection.
Cross-Platform: Build once, run everywhere. PWAs work on any device with a modern browser.
No Need for App Store Approval: Unlike native apps, PWAs don’t require approval from app stores, so you can get your app into users’ hands faster.
Lower Development Costs: Since you only need to write one codebase, development and maintenance costs are reduced.
Push Notifications: PWAs can send push notifications to re-engage users.
Improved Performance: Thanks to caching, PWAs load faster and offer a smoother experience.
Cons of PWAs:
Limited iOS Support: While PWAs work well on Android, iOS support is still limited, especially with Safari. For instance, features like push notifications and background sync are not fully supported on iOS.
Restricted Access to Device Features: Native apps have full access to device capabilities like Bluetooth, GPS, and camera APIs, while PWAs have limited access.
Browser Dependence: PWAs are dependent on the user’s browser to run. If a user is using an outdated browser, your PWA may not work as intended.
Not Suitable for Heavy Apps: For highly complex apps (like video editing software), native apps may still be the better option due to performance and feature limitations of PWAs.
Avoid Common PWA Mistakes
While building a PWA can be straightforward, there are some common pitfalls that developers often fall into. Here’s how to avoid them.
1. Forgetting HTTPS:
PWAs require secure connections (HTTPS) to function properly. Skipping this step will prevent your PWA from working as expected.
Solution: Always deploy your PWA over HTTPS. Services like Let’s Encrypt offer free SSL certificates to make this process easier.
2. Not Testing on Multiple Devices:
A PWA that works perfectly on your desktop browser might not behave the same way on a mobile device. PWAs are meant to be cross-platform, so you need to test on different browsers and devices.
Solution: Use browser developer tools to simulate mobile devices, or simply test on physical devices to ensure consistent performance.
3. Inefficient Caching:
Caching everything can lead to performance issues, especially if the cache becomes bloated with unnecessary files. Over-caching can also result in users not receiving the latest content.
Solution: Only cache what’s necessary, and make use of cache versioning to control updates and avoid serving outdated resources.
4. Ignoring Service Worker Updates:
If your service worker is not set up to update itself correctly, users may get stuck with an outdated version of your app, leading to bugs or missing features.
Solution: Use the skipWaiting()
method in your service worker to activate the new version as soon as it’s available.
self.addEventListener('install', event => {
self.skipWaiting();
});
5. No Fallback for Older Browsers:
Not all browsers fully support PWAs, especially older versions of Safari or Internet Explorer. This can result in a poor experience for some users.
Solution: Provide a fallback experience for users on outdated browsers. You can detect whether the user’s browser supports service workers and display a warning message or provide alternative functionality.
Conclusion: Start Building Your PWA Today!
Progressive Web Apps are an exciting evolution in the world of web development. They combine the best aspects of native apps and websites to offer fast, reliable, and engaging experiences for users. By following these five steps—building a simple web app, adding a web app manifest, registering a service worker, implementing caching strategies, and testing your app—you’re well on your way to creating a fully functional PWA that users can install and access offline.
With so many big names (like Twitter, Pinterest, and Starbucks) jumping on the PWA bandwagon, now’s the time to consider building one for your project or business. If you want to stay ahead of the curve and provide your users with the best possible experience, PWAs are the way to go.
Still on the fence? Try building a small-scale PWA for a side project or a landing page, and you’ll see the benefits firsthand. The beauty of PWAs is that they’re scalable—you can start small and add more features as you go.
Ready to give it a go? I’d love to hear about your experiences building PWAs—drop a comment below, and let’s start the conversation!